Python Tuples and Tricks By albro
hive-169321·@albro·
0.000 HBDPython Tuples and Tricks By albro
<center>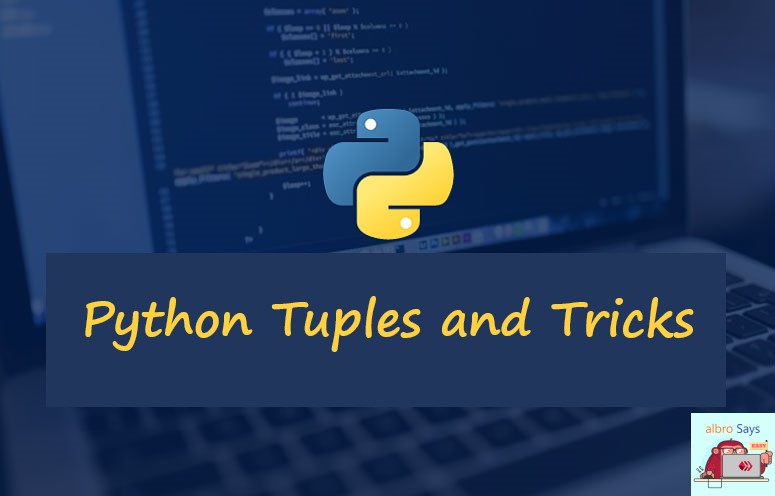</center> <p>A tuple in Python is an ordered and immutable set of values. With the help of tuples, we can store several values of different data types in a variable and perform operations on them. In this post, I will talk about the <strong>tuple data structure in Python and its tricks</strong>.</p> <p>Tuple is a type of <strong>collection</strong> that can store an unlimited number of values.</p> <p>In terms of definition and usage, it's similar to <a href="https://peakd.com/hive-169321/@albro/python-list-and-tricks-by-albro">list in Python</a>. Of course, they have clear differences that we will get to know later. If you're not familiar with lists, I suggest that you definitely learn to work with lists because they are very useful.</p> <p>Unlike lists, values defined as tuples are <strong>immutable</strong>. That is, when we create a tuple with three values, we cannot change their value and number.</p> <h3>Definition of tuple in Python</h3> <p>To define a tuple in Python, open and close parentheses are generally used at the beginning and end. We separate the desired values with a comma (<code>,</code>).</p> <p>Below I have created a tuple with five values.</p> <pre><code>my_tuple = (217, "albro", 23.471, 9, "Hive")</code></pre> <p>As you can see, each tuple element can be of a different data type. In this example, I used numbers and <a href="https://peakd.com/hive-169321/@albro/python-string-and-tricks-by-albro">strings in Python</a>; But we can also use a list object or another tuple!</p> <p>Tuple members are not necessarily different! For example, below we put three numbers in a tuple.</p> <pre><code>tpl = (17, 14, 20)</code></pre> <p>If we put an open and close parentheses for a variable, we have created an empty tuple.</p> <pre><code>empty_tuple = ()</code></pre> <p>Be careful that if our tuple contains only one element, we must also put a comma after the element definition.</p> <pre><code>test = (27,)</code></pre> <p>If we don't put the sign <code>,</code> after the single member in the tuple, that variable is no longer a tuple and its type will be of the same type as the member element.</p> <p>In the image below, I have put the name of the hive as a string in parentheses and assigned it to the <code>test</code> variable. When I check the variable type, we see that <code>string</code> is recognized.</p> <center></center> <h3>Access to tuple elements</h3> <p>Similar to the list or string that has an <code>index</code> to access its elements, we use indexes to access tuple elements in Python.</p> <p>To access the <code>nth</code> element, it is enough to write the name of the tuple and write the index number of the desired house in the brackets (<code>[]</code>).</p> <p>The first member of a tuple has index number zero (<code>0</code>) and its last member (<code>nth</code>) has index <code>n-1</code>.</p> <p>Suppose we have the following tuple.</p> <pre><code>tpl = ("albro", "ecency", "xeldal", "ocdb", "mightpossibly", "leo.voter")</code></pre> <p>To access the third member of this tuple, we must call index <code>2</code> or <code>-4</code>; As follows:</p> <pre><code>print( tpl[2] )<br /> print( tpl[-4] )</code></pre> <p><strong>Range access to elements</strong></p> <p>Similar to other collection type data, <strong>slicing operations</strong> can be performed on tuples. For this, it is enough to define the range of indices with the sign <code>:</code> during the call.</p> <pre><code>tpl = ("albro", "ecency", "xeldal", "ocdb", "mightpossibly", "leo.voter") print( tpl[1:5] )<br /> # Result: # ('ecency', 'xeldal', 'ocdb', 'mightpossibly')</code></pre> <p>In the code below, you'll see more examples of accessing tuple members in these same ways.</p> <pre><code>print( tpl[2:-1] ) # ('xeldal', 'ocdb', 'mightpossibly')<br /> print( tpl[-5:-1] ) # ('ecency', 'xeldal', 'ocdb', 'mightpossibly')<br /> print( tpl[-4:4] ) # ('xeldal', 'ocdb')</code></pre> <h3>Tuple editing in Python</h3> <p>Tuples are <strong>immutable</strong>. Therefore, we will not be able to change the members of a tuple like lists!</p> <p>In the image below, I have defined a tuple and I want to change the value of its second house. As you can see, I got a <code>TypeError</code>.</p> <center>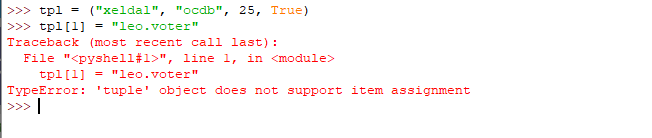</center> <p>The most fundamental difference between a list and a tuple in Python is that because tuple is immutable in Python, we cannot change its values or increase or decrease their number.</p> <p>but don't worry! In the following, we will learn the method by which we will be able to have a new tuple with new members using the current values.</p> <p><strong>Add new member to tuple</strong></p> <p>The addition operator (<code>+</code>) is used to join two tuples together. When using this operator, the first and second tuple values are put together and a new tuple is created.</p> <p>Note that the previous tuples are neither deleted nor changed, only a new tuple will be created with their members.</p> <p>Suppose we have <code>tuple1</code> and <code>tuple2</code>. In the following code, we combine these two tuples with the addition operator and create <code>tuple3</code>.</p> <pre><code>tuple1 = ( "Test", "Element" ) tuple2 = ( 11, 37, 23 ) tuple3 = tuple1 + tuple2</code></pre> <p>The result of concatenating two tuples is as follows.</p> <pre><code>print( tuple3 ) # ('Test', 'Element', 11, 37, 23)</code></pre> <p><strong>Remove tuple elements</strong></p> <p>Just as it is not possible to change an element in a tuple, it is also not possible to delete an element. We can only delete the entire tuple.</p> <p>To delete a tuple in Python, we use the <code>del</code> keyword before its name. This will remove all members of the tuple and the tuple itself.</p> <pre><code>tpl = ("albro", "xeldal", "mightpossibly") # Some Codes... del tpl</code></pre> <p>Note that if I call it after removing the tuple, I get an error similar to the one below. Because there is no such variable in the program.</p> <center>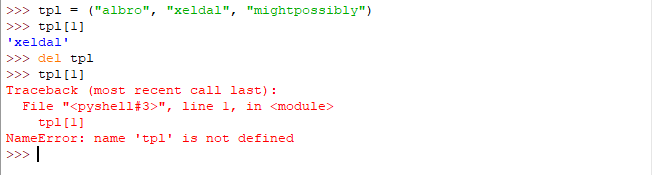</center> <center></center> <p>With the help of operators and key expressions, we can work with tuples more professionally. In the following, I'll discuss four of them.</p> <p><strong>Tuple size with <code>len</code> function</strong></p> <p>The <code>len()</code> function takes a tuple as input and returns the number of elements in it.</p> <pre><code>tpl = ("xeldal", "ocdb", "leo.voter", "steemstem") len( tpl ) # 4</code></pre> <p><strong>Concating tuples to each other</strong></p> <p>We concatenate two tuples to add a new element to the tuple. This work is called concat.</p> <p>For this purpose, between two or more desired tuples, we use the plus sign (<code>+</code>).</p> <p>By doing this, the tuples are concatenated and a larger tuple containing their members is created.</p> <pre><code>tuple1 = ( "Test", "Element" ) tuple2 = ( 11, 37, 23 ) tuple3 = tuple1[1:] + tuple2[:-1] print( tuple3 )<br /> # Result: # ('Element', 11, 37)</code></pre> <p><strong>Iterate tuple elements in Python</strong></p> <p>Suppose you have a tuple with two elements. You want to iterate over these elements and put them in a new tuple.</p> <p>For this, we use the mathematical multiplication sign (<code>*</code>). It is enough to multiply the tuple by the desired number of repetitions. With this, we will have a new tuple with arbitrary repetition of the previous elements.</p> <pre><code>tpl = ("xeldal", "mightpossibly") print( tpl*3 )<br /> # Result: # ('xeldal', 'mightpossibly', 'xeldal', 'mightpossibly', 'xeldal', 'mightpossibly')</code></pre> <p><strong>Checking the existence of an element in a tuple</strong></p> <p>If we want to check the existence of a value in the tuple, we will use the in keyword. We put the desired value on the left side and our tuple on the right side.The output of this expression will be <code>True</code> or <code>False</code>; So it can be used in <a href="https://peakd.com/hive-169321/@albro/python-condition-structure-by-albro">Python's conditional structures</a> as well.</p> <pre><code>tpl = (17, 25, 32, 37, 42, 50) print( 32 in tpl ) #True print( 35 in tpl ) #False</code></pre> <h3>Summary</h3> <p>In this post, we learned about tuples in Python. We specify the tuple with parentheses. Its elements and length are immutable, and if we want to add a new member to it, we technically need to create a new tuple.</p>
👍 bgmoha, pars.team, elector, goldfoot, botito, tobor, hadaly, dotmatrix, curabot, chomps, freysa, lunapark, weebo, otomo, quicktrade, buffybot, hypnobot, psybot, chatbot, psychobot, misery, freebot, cresus, honeybot, dtake, quicktrades, droida, mproxima, taradraz1, pibara, incublus, chessbrotherspro, misterlangdon, doudoer, eumorrell, jrjaime, fernandoylet, frankrey11, vjap55, cyprianj, eniolw, larsito, rosmarly, oabreuf24, nazom, raca75, philipp87, jrevilla, lemouth, steemstem-trig, steemstem, dna-replication, minnowbooster, howo, aboutcoolscience, robotics101, stemsocial, edb, bhoa, alexdory, crowdwitness, zeruxanime, curie, techslut, dhimmel, oluwatobiloba, mobbs, helo, tsoldovieri, postpromoter, kenadis, r00sj3, intrepidphotos, emiliomoron, melvin7, gadrian, deholt, plicc8, walterjay, valth, alexander.alexis, abigail-dantes, pab.ink, temitayo-pelumi, pboulet, apokruphos, samminator, aidefr, madridbg, sco, fragmentarion, noelyss, lamouthe, sustainablyyours, geopolis, francostem, de-stem, stem.witness, windail1, the-grandmaster, beta500, enzor, satren, doctor-cog-diss, cnfund, maxdevalue, meritocracy, dcrops, t-nil, superlotto, cleanplanet, steemstorage, federacion45, otp-one, laruche, cleanyourcity, cliffagreen, sarashew, aicu, felt.buzz, fotogruppemunich, justyy, revo, thesoundof, therealwolf, merlin7, brianoflondon, roamingsparrow, tanzil2024, princessmewmew, neneandy, podping, smartsteem, djlethalskillz, punchline, arunava, kylealex, cloh76, dynamicrypto, meno, braaiboy, steemwizards, cakemonster, baltai, brotherhood, takowi, photohunt, citizendog, bilpcoinbpc, traderhive, yixn, mamalikh13, greddyforce, neumannsalva, zyx066, fineartnow, pandasquad, empath, investingpennies, utube, cheese4ead, thelittlebank, irgendwo, rt395, thelordsharvest, sportscontest, fantasycrypto, bitrocker2020, communitybank, metroair, aabcent, reverio, musicvoter2, sbtofficial, sunsea, qberry, hiveonboard, detlev, ahmadmangazap, seinkalar, armandosodano, kristall97, steemcryptosicko, aries90, gohive, steveconnor, iansart, gunthertopp, stayoutoftherz, voter002, enjar, jayna, the-burn, tfeldman, diabonua, bflanagin, cryptofiloz, humbe, talentclub, therising, juancar347, oscarina, goblinknackers, jacuzzi, steemean, qwerrie, trenz, adrianalara, xeldal, rmach, mcsvi, adol, enki, ibt-survival, xxeldal, elevator09, forykw, sanderjansenart, mafufuma, robibasa, potsuperiya, carilinger, atheistrepublic, dondido, lightpaintershub, lordvader, jerrybanfield, uche-nna, walterprofe, mammasitta, dandays, vaultec, nfttunz, eric-boucher, robertbira, lk666, nicole-st, imcore, flatman, stea90, gamersclassified, roelandp, ydaiznfts, spiritabsolute, aioflureedb, pewsplosions,